What are Zombie Accounts? Risks & How to Fix Them
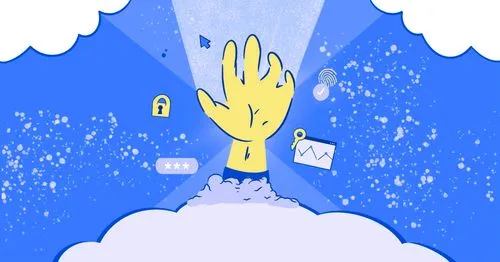
What are Zombie Accounts?
Zombie accounts are user accounts that linger in systems and applications without active use or proper management. These usually either belong to employees who have left the organization or were created for specific purposes that are no longer relevant. Over time, these idle accounts become potential vulnerabilities, exposing organizations to a variety of risks.
How are Zombie Accounts Created?
Zombie accounts often result from oversight, employee turnover, or poor management. When accounts aren't deactivated after an employee leaves or a project ends, they become dormant, posing data exposure risks.
Without strong security measures like multi-factor authentication, these accounts can be vulnerable to unauthorized access. Implementing security protocols and regular account management is key to preventing zombie accounts from becoming potential security risks.
Factors Contributing to Zombie Account Creation
The Risks of Zombie Accounts
The risks of zombie accounts go beyond being inconvenient; they can lead to compliance issues, financial costs, and serious security problems. Understanding these risks is key to building strategies that protect an organization’s digital security.
- Compliance risk: One of the primary risks associated with zombie accounts is compliance failure. Many industries and organizations are subject to strict regulations governing user access and data protection. Failure to manage and deactivate accounts in accordance with these regulations can lead to severe consequences, including legal actions and financial penalties.
- Financial risk: Zombie accounts can contribute to financial risks in various ways. Unused software licenses tied to inactive accounts represent wasted resources, and organizations may inadvertently continue paying for these licenses. Moreover, unauthorized access through dormant accounts can lead to financial losses due to potential data breaches or malicious activities.
- Security risk: Security is a paramount concern when dealing with zombie accounts. Forgotten accounts become attractive targets for malicious actors seeking unauthorized access to sensitive information. These accounts may lack the latest security updates, making them vulnerable entry points into an organization's systems.
How to Identify Zombie Accounts and Delete Them
Identifying and eliminating zombie accounts is important to preserving security and preventing unauthorized access. By following these targeted steps, organizations can efficiently detect and delete dormant accounts before they become security risks:
- Review inactive accounts: Regularly scan for accounts with extended inactivity, using the last login date to identify potential zombie accounts.
- Monitor login activity: Track login patterns to detect unusual access attempts or prolonged inactivity, indicating dormant accounts.
- Cross-reference HR records: Coordinate with HR to ensure all accounts that belonged to departing employees are deactivated promptly, preventing any accounts from lingering post-exit.
- Audit permissions and roles: Regularly review account roles and permissions, deactivating those that no longer align with current responsibilities or have been left unused.
- Use automated tools: Use automated IAM tools to efficiently flag and disable inactive accounts, reducing manual effort and the risk of oversight.
Best Practices for Preventing Zombie Accounts
As we explore zombie accounts and the risks they bring, the key to stopping them is to follow best practices. Prevention is critical for strong cybersecurity, and clear steps can prevent zombie accounts from spreading.
Effective offboarding, role-based access controls, and regular account audits are important practices that help strengthen an organization's defense against this threat.
Account Deactivation Workflow
In cybersecurity, creating an effective account deactivation process is key to preventing zombie accounts. This process goes beyond just deactivation; it ensures departing employees' access is fully revoked across all systems and applications.
Central Repository for Software Licenses
Maintaining a central repository for software licenses helps organizations keep track of active and inactive licenses. Regularly auditing and updating this repository ensures that unused licenses tied to zombie accounts are identified and reallocated, preventing unnecessary expenditures.
Role-Based Access Control (RBAC)
Adopting Role-Based Access Control (RBAC) helps organizations assign and manage user permissions based on job roles. This ensures that employees have the necessary access for their responsibilities and nothing more. When roles change or employees leave, access permissions can be updated accordingly, minimizing the risk of zombie accounts.
SaaS Application Ownership Framework
In the era of cloud computing and Software as a Service (SaaS), establishing a clear ownership framework for applications is essential. Assigning responsibility for each SaaS application ensures that someone is accountable for user access, reducing the likelihood of zombie accounts slipping through the cracks.
Subscriptions Approval and Renewal Policies
Implementing strict policies for subscription approvals and renewals helps organizations maintain control over their accounts. Regularly reviewing and approving subscriptions ensures that only necessary and actively used services are retained, preventing the proliferation of zombie accounts tied to unused subscriptions.
Regular User Account Reviews
Conducting regular reviews of user accounts is a proactive measure to identify and address zombie accounts promptly. These reviews can be part of a routine security audit, enabling organizations to detect dormant accounts and take corrective actions.
Revocation of Access
Establishing clear procedures for access revocation is crucial for preventing unauthorized access through zombie accounts. When an employee leaves or changes roles, their access should be promptly revoked to mitigate security risks.
Strong Password Policies
Enforcing strong password policies adds an additional layer of security to user accounts. Regularly updating passwords and implementing multi-factor authentication safeguards against unauthorized access, reducing the risk of zombie accounts being exploited.
Conclusion
In today's digital world, managing user accounts is key to security and compliance. Understanding the risks of zombie accounts and using best practices is essential. By setting up effective workflows, access controls, and regular reviews, organizations can protect themselves from these hidden threats. Proactive steps not only improve security but also help with efficient resource use and compliance. Stay alert, stay secure.